Homework 2: Arrays & Lists
Due: Thursday, February 21, Friday, February
22, before 9:00 pm
Instructions: Your solutions to the following problems must be typed, converted to PDF, and submitted on Blackboard. It is okay to discuss concepts underlying the problems with other students, but all final solutions must be your own work.
All solutions must be typed, converted to PDF, and submitted on Blackboard. No other format is acceptable. Scans or photos of hand-written solutions will not be accepted.
Question 1
In Project 1, we used compressed sparse row (CSR) format to store the adjacency matrix of a graph. Another data structure that could be used is an adjacency list (AL), an array of linked lists, one per vertex. The linked list for vertex v stores the neighbors of v (and corresponding edge weights, if any).
Following is a small example of a graph and its adjacency list. Note that the neighbor lists are not ordered by increasing column index.
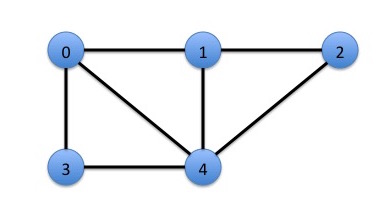
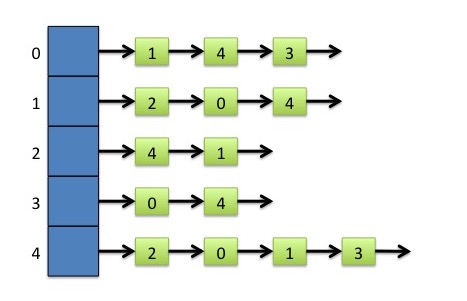
In the following, assume that there are n vertices, m_head is an n-long array of Node pointers with each entry initialized to NULL, and the Node structure consists of an integer column index ci and a Node pointer m_next. Graphs are unweighted, so there is no need to store edge weights.
- Describe in pseudocode the procedure for adding an edge to the graph. Your procedure should be correct whether or not some edges have already been added. Within each list, nodes do not need to be stored in order of increasing column index.
- Compare the memory requirements for CSR and AL as functions of n, the number of vertices, and m, the number of edges.
- For which data structure (CSR or AL) is insertion of an edge faster? Suppose we added the requirement that the linked lists for AL must be stored in order of increasing column index; which would be faster then? Explain your answers!
- Give a technical reason why you might prefer one of the two data structures over the other. Justify your answer. There's no single right answer to this question.
Question 2
In Project 1, we required that the data for each row of the adjacency matrix be stored in order of increasing column index within the m_nz and m_ci arrays. This wasn't just to make the project harder!
It is common to perform linear algebraic computations such as matrix powers or matrix-vector products with an adjacency matrix. Suppose we have an n-by-n numeric matrix A and a n-long vector x (a mathematical vector, not an STL vector). The matrix-vector product produces an n-long vector y for which the ith element, yi is given by the formula
Note: This is the math description, using one-based indexing.
- Write the implementation of a function Graph::matVec(int *x, int *y) such that the call G.matVec(x, y) computes the matrix-vector product of the adjacency matrix of G with the integer array x and writes the output in the integer array y. You may assume that the calling procedure has correctly allocated x and y as n-long integer arrays.
- How would the implementation of matVec() have to change if the data for each row in m_nz and m_ci were not stored in order of increasing column index?
Question 3 (Adapted from Exercise C-3.3)
Let A be an integer array of length n ≥ 2 containing the integers 0 to n-2, with exactly one value repeated. Describe an algorithm to determine which value is repeated in linear time (time proportional to n). Provide pseudocode and a written explanation.
Extra Credit
Four computer scientists from UMBC are trying to get to a conference in Princeton, NJ. They have two unrestricted train passes: anyone of them can use the passes on any train between the BWI train station and the Princeton train station, in either direction. They decide that two of them should ride to Princeton, one should return to BWI with the passes, then two more go to Princeton, and one returns with the passes, etc., until they are all in Princeton. Unfortunately, some of them are extremely picky travelers:
- The first is the least picky and is willing to take any train. The high-speed train between BWI and Princeton takes one hour in either direction.
- The second is afraid of the high speeds on the fastest trains, but will take a standard train. The standard train between BWI and Princeton takes two hours in either direction.
- The third insists on stopping for a cheese steak whenever they pass Philadelphia. All the trains pass through Philadelphia. The trip between BWI and Princeton, stopping in Philadelphia for a cheese steak, takes five hours in either direction.
- Finally, the fourth traveler also always stops for cheese steak and insists on visiting the Franklin Institute whenever they pass through Philadelphia. The trip between BWI and Princeton, stopping in Philadelphia for a cheese steak and to visit the Franklin Institute, takes 10 hours in either direction.
Whenever any two travel together, they can only make the trip as quickly as the slower of the two. For example, if person 1 and 4 travel together, it will take 10 hours to get to Princeton. What is the least amount of time it will take for them all to get to Princeton? In what order should they travel to achieve the minimum time?