##Chapter 9 - Web Frameworks
###Introduction
Almost all new development for distributed systems today is based around the web infrastructure of using HTTP to access web resources and web services. Creating presentation tier clients with web-based programming frameworks for this infrastructure helps the architects and developers to effectively and quickly build, test, and deploy these applications.
We will survey web-based frameworks in this chapter for major implementation technologies and do some programming exercises using PHP to make the discussion more concrete. We will also take a brief look at a new XML vocabulary, scalable vector graphics (SVG).
The four tier web infrastructure is shown in figure 9.1 with the logical presentation tier highlighted as where these web-based frameworks are focused at delivering web applications. The frameworks involve all layers, however, in that the frameworks use javascript in the web browser, web servers to deliver content, application server technology such as PHP for server-side processing, and databases for maintaining state.
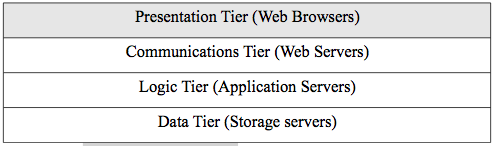
Figure 9.1. The web infrastructure.
Software frameworks are generic, partially written applications for a specific application architecture that make creating new applications quicker and easier. The frameworks offer a large collection of libraries that take care of all the routine programming tasks and leave the programmer to implement the custom functions that are required for the new application. This makes programmers much more productive, but removes flexibility. So as long as the needed application follows the patterns given by the framework, it works well. If a new pattern is needed, the programmer might well be better advised to program from scratch. Web-based applications are well suited to a collection of design patterns that are implemented in web frameworks. Most web frameworks have integrated development environments (IDEs) that are used to easily access the web framework libraries while programming.
Design patterns are an influential concept in software engineering that represent reusable solutions to a commonly occurring programming and software design problems. It is not code but a description of the pattern. Programmers use code to implement the design pattern. Web frameworks are code that implement proven, relevant design patterns.
Design patterns gained popularity in computer science after the book Design Patterns: Elements of Reusable Object-Oriented Software was published in 1994 by the so-called "Gang of Four" (Gamma et al.). A very common design pattern used in web frameworks is the model, view,
controller (MVC) pattern which separates interface, logic, and data models so that code developed with this pattern is more easily maintained, tested, and developed. Figure 9.2 shows the basic MVC pattern.
The general operation of MVC can be described as follows. A web browser makes a request to a web server with a nice looking URL as we defined them earlier for REST web services. The routing layer associated with the web framework translates the nice URL structure to call the specific web applications offered by the controller. The controller gets data from the model for processing. The model is responsible for getting data from the database and creating objects because web frameworks are typically implemented in object-oriented languages and cannot directly operate on relational databases. The controller program participates in a SOA and so can also request SOAP or REST web services. The controller then instantiates a template view for the results of the data processing. This view is usually a web page that is returned to the web server by the controller and the web server returns it to the requesting client. The view templates usually use technology like PHP (or JSP or ASP) that we covered earlier where programming code can be mixed with HTML in a template.
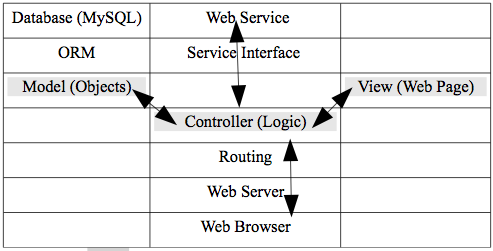
Figure 9.2. MVC.
Another design pattern used by web frameworks is object relational mapping (ORM). In the MVC pattern described above we saw that the model must get data from the database. The ORM layer is between the model and the database and creates a virtual object database that can be used from within the object-oriented programming language. It also uses application (object) caching to improve performance.
There are hundreds of web frameworks in many different programming languages. We will survey only a few of them and concentrate on an example using PHP since we studied that previously. We will classify them according to the three major implementation technologies of J2EE (java), .NET (Microsoft), and web scripting environments.
There are many web frameworks that use the java web application server technology. A java web application must run in a servlet container that communicates with a web server via the java servlet API. Servlet containers are also called web containers. Java servlet containers typically run as a process that creates a new thread for every request.
The most recent and commonly used framework for java is java server faces (JSF) which uses the MVC pattern. The structure of JSF is shown in figure 9.3.
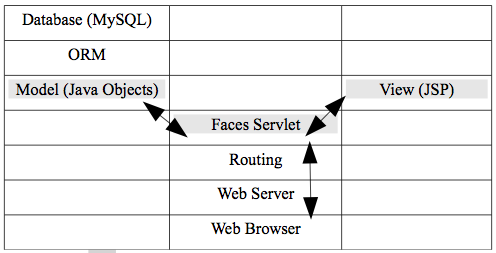
Figure 9.3. JSF.
The faces servlet is the controller that does processing using java objects and instantiates views with Java server pages (JSP) that contain JSF tags. JSF tags are a special tag library that make programming simpler for common web scenarios. There are many IDEs that support JSF.
A common one is NetBeans. Java web application servers can run on almost any operating system.
ASP.NET is an MVC web framework from Microsoft that builds on the .NET component model and uses programming languages such as C#. All the same concepts apply. The web browser requests a page (with a .aspx extension)
and the routing layer converts the URL to an action in the front controller. Since C# is an object-oriented language, there is an ORM layer for the model. Views are created with active server pages (ASP)
that allow code to be mixed with HTML but the code is executed server-side. The ASP.NET framework is highly optimized for Microsoft server environments and makes programmers very productive with the Microsoft Visual Studio IDE, but of course, it can only be used with the Microsoft operating system.
There are many frameworks based on web scripting languages. A few examples are (where the framework name is followed by the programming language in parentheses):
- Ruby on rails (Ruby)
- Express (server-side Javascript)
- Django (Python)
- CodeIgniter (PHP)
Ruby on rails (ROR) has been very influential and has influenced the design of many other web frameworks that use other scripting languages.
The web frameworks for scripting languages are all MVC, use object-
oriented programming languages and follow the same patterns we have already seen. We will look at the open-source PHP framework CodeIgniter (http://codeigniter.com/) in detail since we have PHP available to us on gl. It is a simple framework inspired by ROR. It has very good on-line documentation and tutorials. As with most of these frameworks, we will have to do very little actual programming since the framework does most of the work. You should read the introduction section of the documentation and watch (and implement) the Introduction to Codeigniter 9 minute video that is available on that web site. This will show the basics of how the codeigniter (CI) framework works without using a database (be sure and read the notes on the syllabus first). You use data structures directly in the controller instead.
A few details about PHP and the gl environment are needed before you start working with CI. Firstly, the point of this exercise is not to make you an expert programmer. The point of this exercise and those below are to make the concepts associated with frameworks concrete, just as in chapter 3. It is not necessary to be an experienced programmer to complete these exercises because the code is given to you. Just reading about the concepts, however, does not result in any real understanding.
Unfortunately, much of your other university coursework does not understand this! Doing the exercises will help you internalize the concepts which you need to understand in order to make decisions about designing and evaluating technological solutions.
CI uses object-oriented PHP. The main thing you need to understand the code given to you is that PHP classes are created and inherit from superclasses using the syntax:
`< ?php class Student extends Person {...} ?>`
where the class code goes inside the curly braces and Person here is the superclass. Recall from your previous programming classes that objects have properties and methods that will be defined here. PHP uses the arrow notation (->) to refer to an object's methods or properties as in:
`$myUser->verifyPassword(); or return $this->name;
`
where $this is a built-in variable and refers to the current object -
typically from inside a class.
The environment on gl has a few shortcomings... It only supports PHP4 which is not a good deal since PHP5 is much better. But we do what we have to and CI was chosen in large part because it supports PHP4 (but we can't use the most current version since PHP4 was recently depreciated in CI version 2). Also, gl does not have the Apache API module rewrite installed and so we will not be able to create really nice- looking URLs using an .htaccess file. This is explained in the first CI video. None of these things really effect the lessons we need to learn, but are annoying!
You should read the on-line installation instructions, but I give you customized ones for gl below. The installation basically just requires copying some files. The installation steps are:
- Use the wget command from the gl command line to copy the zip file from the CI download section to your www directory. Download the CI v 1.7.3. This way, you will have the file on gl with no need to re-upload.
- Use the unzip command to unzip CI (I have placed links to documentation for relevant unix commands on the on-line course syllabus). You will end up with a directory called CodeIgniter_1.7.3, but you may want to rename it to CI for convenience.
- Look in the CI directory and notice that you have the index.php front controller that receives requests and that inside the system/application directory, you see all the framework directories for controllers, models, and views where you will place your own code for the exercises. You can either edit the files directly on gl, or edit them on your PC and use scp to upload them.
Read the following sections of the CI documentation (for 1.7.3 which is included in your download):
- Introduction
- all subsections
- General Topics
- CodeIgniter URLs
- Controllers
- Views
- Models
- Helpers
- Caching
- Class and Helper References
- Array Helper
- URI Class
- HTML Helper
You also need to setup your MySQL database. Your gl account has been configured with access to the database already. Use the following steps to set it up:
1. ssh to your gl account.
2. type the command: `mysql -u username -p`
where you use your own username. You will be prompted for your password and use your username again. When they first make your accounts, that is the way they configure them - with the username as the password (very insecure so now change your password with the mysqladmin GUI).
3. You will now see the mysql prompt where you can type mysql commands or SQL commands.
4. You can get out of mysql by typing `\q` and you will return to the gl prompt.
5. CI will do most of the database work for you using scaffolding, but you must understand the following:
- The host name for the database server is studentdb.gl.umbc.edu. You will need to enter this in code in a configuration file for the exercise. Mysql is on a different host than gl.
- In mysql, one can normally create any number of databases with any number of tables in each database. This is not true for our student implementation. You only have one database and it has your username as the name. You cannot create additional databases or change the name of the single database. You can create any number of tables within your one database, however. This will work fine for the exercise.
- You can see and manipulate databases with the mysqladmin web-based interface at: http://webadmin.umbc.edu/mysqladmin/. You will be using it later to create database tables. You have to go through the vpn (vpn.umbc.edu) from off campus.
We will now take a look at an XML vocabulary for graphics.
###SVG
SVG is an XML vocabulary for describing 2-dimensional vector graphics.
It is a W3C specification that began in 1999. The current version is SVG 1.1. Vector graphics is the use of geometrical primitives such as points, lines, curves, and shapes or polygon(s), which are all based on mathematical equations, to represent images in computer graphics. So vector graphics can be resized to different resolutions (the scalable part) without resampling or image degradation. Raster graphics on the other hand, represents images as an array of pixels (a bitmap) and have a fixed resolution that can only be changed by resampling algorithms than add or subtract from the image resulting in degradation. Png, gif and jpg are all examples of raster graphics.
We will use the on-line w3schools tutorial for SVG. Our main goal here is just to see a novel implementation of another XML vocabulary. Since it is a specification that began in 1999, it is a vocabulary defined from a DTD. They have not changed that to XMLSchema in recent versions,
but have added a namespace: http://www.w3.org/2000/svg. Read the short tutorial and play around with the tutorial examples of SVG using the `Try it yourself >>` tool. Note that several of the images in this book are SVG. Figure 8.3,
for example began as an SVG file (repeated here as figure 9.4).
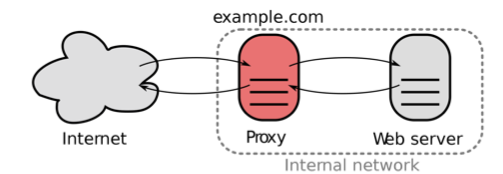
Figure 9.4. Reverse proxy cache (SVG file).
Go to http://en.wikipedia.org/wiki/Proxy_server and find the freely licensed image. Click on the image and see that it is from the file:
Reverse_proxy_h2g2bob.svg. Click on the file link and view the source of the file. It is a complicated file and it is not necessary to understand it all. Notice, however, that it does not use the DOCTYPE,
but does use namespaces. This is actually the recommendation of the W3C - to not use the DOCTYPE and DTD, but to use namespaces (w3schools does not seem to have gotten the message!). This is a peculiarity of the fact that SVG was originally created long ago as an application of XML using a DTD, but DTDs do not handle namespaces. Note that there is a namespace for Inkscape. This is an open-source application for creating and editing SVG files.
Google has recently started indexing all SVG on the web and so now you can do custom searches for SVG. Go to google and do an image search on proxy server. Click on advanced search and choose the SVG filetype. You should see the image in figure 9.4 in the results.
Adobe Flash is currently the most widely used vector graphics and animation format on the web, but it is in a proprietary, binary format that makes interoperability problematic. SVG mixes easily with web services since it is XML. One can send SVG diagrams or animations via SOAP or REST messages. HTML5 supports SVG and its own Canvas drawing technology and many think this will replace Flash. SVG is better for high resolution and Canvas is better for fast animation.
###Chapter 9 Exercises
Do the end-of-chapter exercises for each chapter of the book by following the link in the on-line syllabus.